Generating Apartments
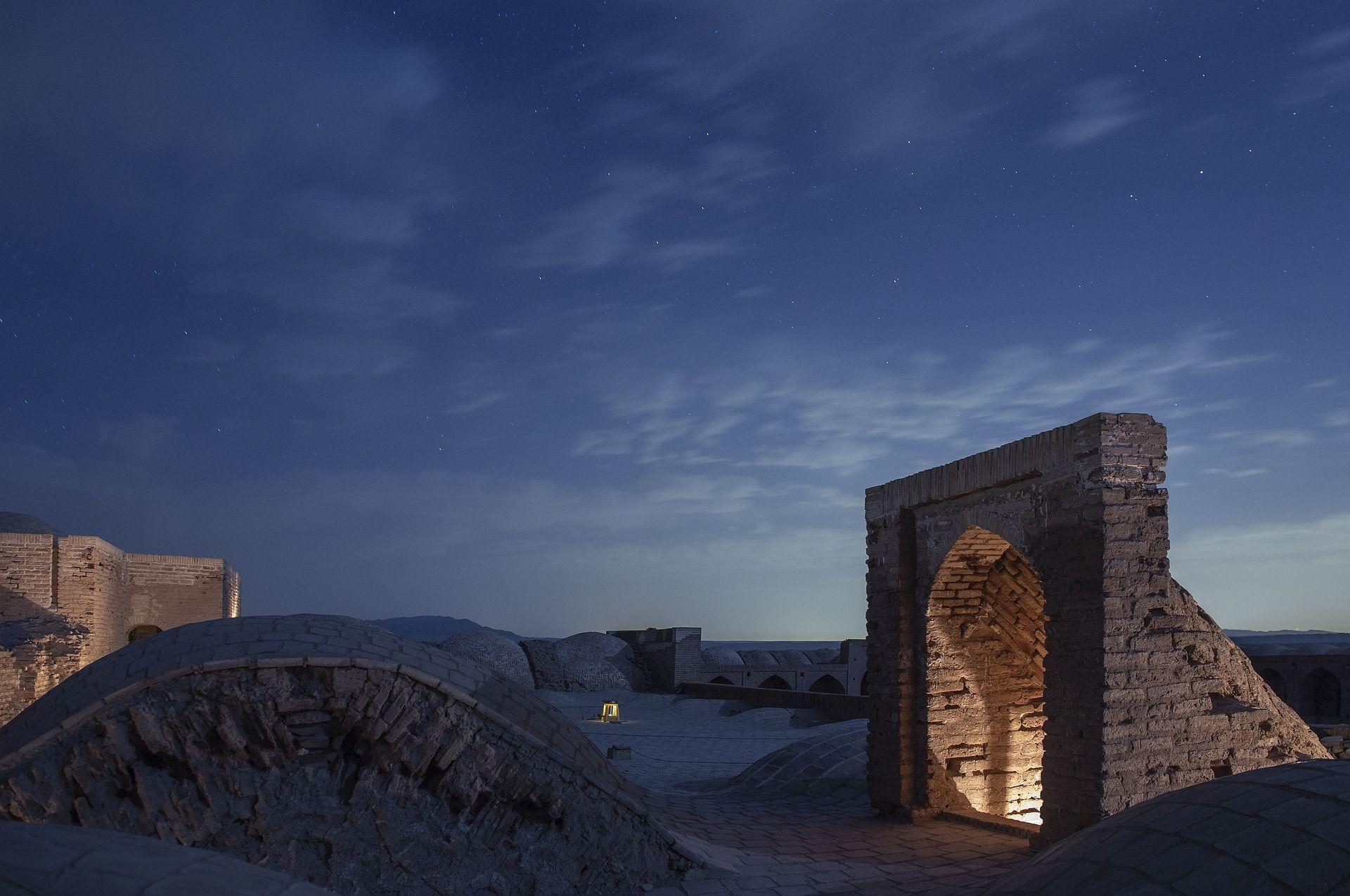
So today I pick up where I left off yesterday. I will figure out my UUID conversion bug and go from there.
TL;DR: It was foreign key ID type mismatch. I'm a fucking idiot sometimes.
I have no idea what could be causing the bug but maybe with a little searching around my code and the internet I will sort it quickly. I know this is something wrong with my query, at least. I built another table to test against and I know I used UUIDs fine for several other things ruling out anything wrong with the PGClient.
So, what then have I fucked up?
INSERT INTO apartment_house (id, name, base_scene, background_id, open_to_visitors, temperature, humidity, energy, max_energy)
values
(
'%s','%s','%s','%s','%s','%s','%s','%s','%s'
);
[apt_id, char_name + "'s Rad Apartment", "BasicApartment", 1, false, 70, 50, 100, 100]
Where apt_id is a string of a UUID4, of course. The error is here, in the ID. I know the field is a UUID field, I know that the same steps worked before to create accounts and characters. So, then; what is the error?
Examining a working query using a UUID4...
INSERT INTO player_accounts_player_account (username, password, email, registration_time, banned, player_id) values ('%s','%s','%s','%s','%s','%s');
[username, hash_password(password), email, Time.get_datetime_string_from_system(), "false", str(UUID.v4())])
I decided to try changing where the query runs, just so I can more easily debug it... in the process I noticed my character creation broke. Actually, the things I fixed when I changed the character sprites are... UN-fixed. Also the server seems to not be receiving the data when I click create character. Also the skin color change no longer works.
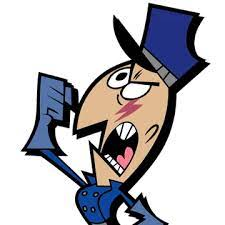
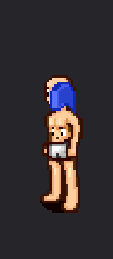
I guess my margins are being undone by godot at some point, probably when I exit, means I gotta set them in code I guess...
That didn't work.
Also the apartment server is no longer receiving the character data when the client sends it... so that's... yeah. Seems like a lot is going wrong lately.
So I fixed the issue with the character creation, somehow a piece of code I have not messed with in a couple weeks has an indent removed. A function was returning early.
Apartments still busted and character creation display is also still quite busted. Sometimes I wonder if there is some force trying to prevent me from making games. Anything else I do seems fine but when I make games I encounter all kinds of problems. Internet bill fuckery, sick cat, sick dog, sick me, power going out, front lawn sink hole, my laptop power circuitry exploding, etc. It's really uncanny.
Curse-monger, I defy thee.
So, instead of fixing the character creation UI bug, which I really aught to get too as soon as I can, I will focus on my apartment creation bug because that crashes my server and directly halts progress.
I really am stumped, it's probably something staring me right in the face, something terribly obvious that I will look back on and slap my forehead so hard my head will look like a baseball mitt.
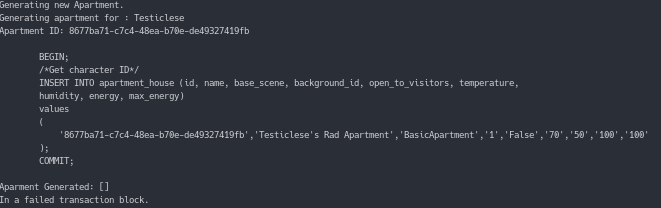

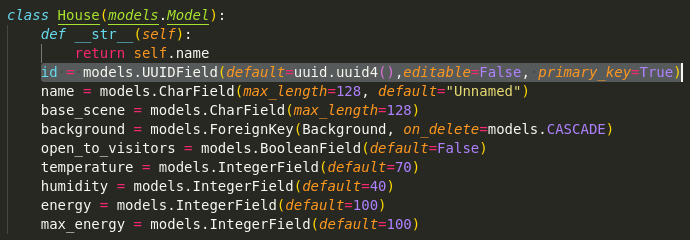

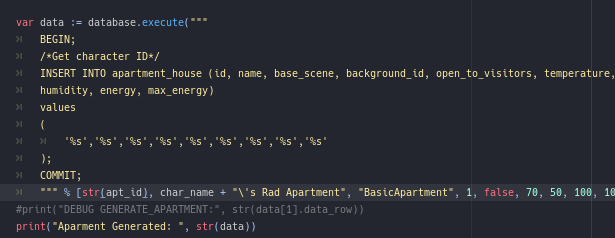
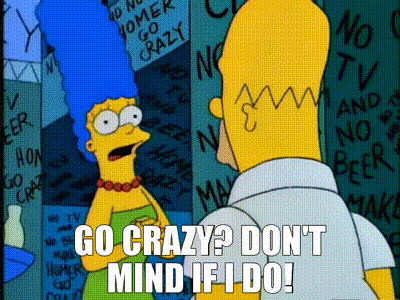
This is the fourth thing I have set up like this, you'd think it'd work fine. There's something here, something I am not seeing. What could it be? How do I debug it? Hmm... I know the type of the UUID is String, I know the DB accepts Strings for the UUIDs because I have tested multiple ways of inputting the dang things.
I know it shouldn't be the PostgreSQL Client because it's doing this stuff several times before we even get to this point without issue.
I know all the data going in is correctly formatted and that the query is formatted correctly. I can run the query that I print out in the terminal client and generate an apartment that way, no problem.
If I paste in the output of that print statement:
INSERT INTO apartment_house (id, name, base_scene, background_id, open_to_visitors, temperature, humidity, energy, max_energy)values('eb98617b-f187-42ae-bd9a-685c4a695353','Home of Testiclese','BasicApartment','1','False','70','50','100','100');
Which is the query it is trying to run...

The hell?
I wish I had any idea why this was happening.

Tested with the apartment_apartment table which has a slightly different setup and still get the same shit. I know it's pointless but I will try restarting the RDBMS.
Actually, before I do that... I am going to see if SOMEHOW there's some fuckery going on in the whitespace. I know there shouldn't be but we're shooting in the dark now so let's see what happens when I remove all the whitespace in the query?
Didn't think so. The error messages have reduced to one, however... so that's new.
Another thing I will try before I go about restarting postgresql is just... putting an int in the UUID space. Fuck it, what could go wrong?
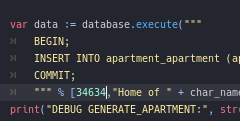
Uhhh, that went through. You wanna run that one by me again?
...and somehow it's a UUID now? WHAT THE HELL IS ANYTHING!?

So, give it a number and it gets converted to a UUID I assume by postgres? Maybe something in the log?
Yeah, it seems to be perfectly happy to take an integer but not a string... though every other table I have UUIDs in I give it a string and have no problems.
Madness.
I miss drinking sometimes.
Okay, let's think about this. I can pass a string no problem up until this point, where I get an error. Now if I pass an INT I get a UUID showing up in it's place. I wonder if I pass the same integer twice will I get the same UUID?


So like, this is supposed to drive me mad.
I give it an INT when it asks for an INT, it works ONCE. I do it again, NO DICE! NOW it wants a STRING again. So I give it a STRING and IT WANTS A FUCKING INTEGER!
WHAT IS THIS MADNESS!?
Some quantum bullshit here, tell you wot.
Restarted Postgres.

Nope.

So I wrapped the UUID in double quotes for... reasons. That worked THIS time. I suspect it will not work next time as I know what happens with double quotes.
YEP.

Just above the apartment generator code I have this:
var char_id = UUID.v4()
# Now we write a bastard of a query. Hang on to your asses.
var data := database.execute("""
BEGIN;
/*Get character ID*/
INSERT INTO character_character (id, name, level, experience, strength, agility,
constitution, intellect, wisdom, charisma, current_health, max_health, current_sp,
max_sp, current_weapon_skillpoints, max_weapon_skillpoints, short_blade, short_blade_xp,
long_blade, long_blade_xp, axe, axe_xp, projectile, projectile_xp, thrown, thrown_xp,
spear, spear_xp, face_sprite, hair_sprite, hair_color, skin_color, gender)
values
(
'%s','%s','%s','%s','%s','%s',
'%s','%s','%s','%s','%s','%s','%s',
'%s','%s','%s','%s','%s',
'%s','%s','%s','%s','%s','%s','%s','%s',
'%s','%s','%s','%s','%s','%s','%s'
);
COMMIT;
""" % [char_id, cn, 1,0,1,1,1,1,1,1,10,10,5,5,6,150,1,0,1,0,1,0,1,0,1,0,1,0,int(cf),int(ch),int(chc),int(csc),cg])
So I know this should work.
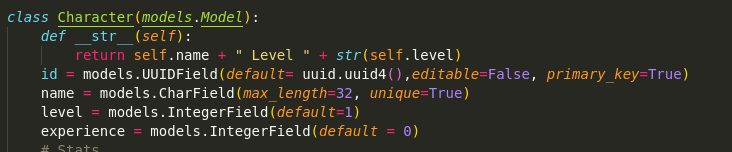
Let's try NO QUOTES because that'll change something meaningful, right!?
That did what I expected it to. I like when things do what I expect, however the only things that are going as I expect them to are errors.

And we're back.

So...
Yeah...
Now what?
You know how I said it was likely something staring me in the face? Something obvious? Yeah, I am what you call... an moran.
Soooooo the bigint error was correct. The problem was that the error output I got from the client in godot was pointing me in the wrong directions. See...
My stupid ass when writing my models didn't use UUIDs for some things I should have and I went to retroactively add them as a field and figured it would be fine. Now, the second query in that function sets the apartment on the player's account.
THAT is a foreign key that IS EXPECTING AN INTEGER because I of course set the bastard up so it's IDs are INTs. GENIUS! Now, let's just go and use my new apartment_house table where I corrected this error. I will point the foreign key at the new table and set the queries to use that table as well. This should fix my error.
Should.

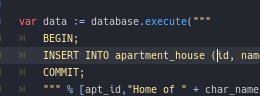
Now to make and run migrations...
Ah, right. It's not able to cast. Uh... I know. I'll delete that migration and fix it with some stack overflow.
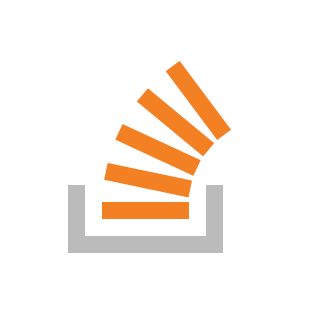
Or not. Seems to be buggered.
Looks like it's time for duct tape solutions. I'll drop apartment_apartment and use apartment_house, House will be the column now. I just need this to be over so I can get back on track. I'll use the apartment for something else later on, I think... I do have a few ideas.
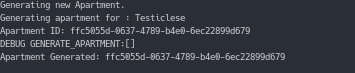
Yep.
That's about right.
But did it really?
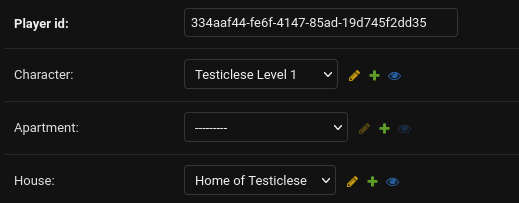
How about over in yon furniture placement table?
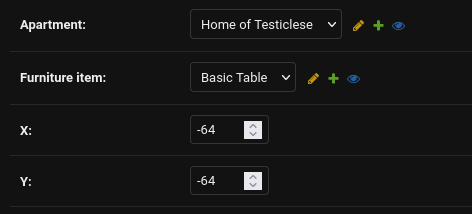
So I spent the entire time debugging the wrong query because the debugger would always highlight the generation query, not the assignment to the player account query... and it didn't generate anything in the apartment table so I didn't think to question it.
Live and learn.
Time to cook dinner, though. I am out of here. What a fuckin' journey.
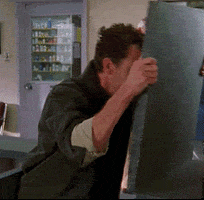