Mantilogs Client Part 1.5 and 2: Displaying API data
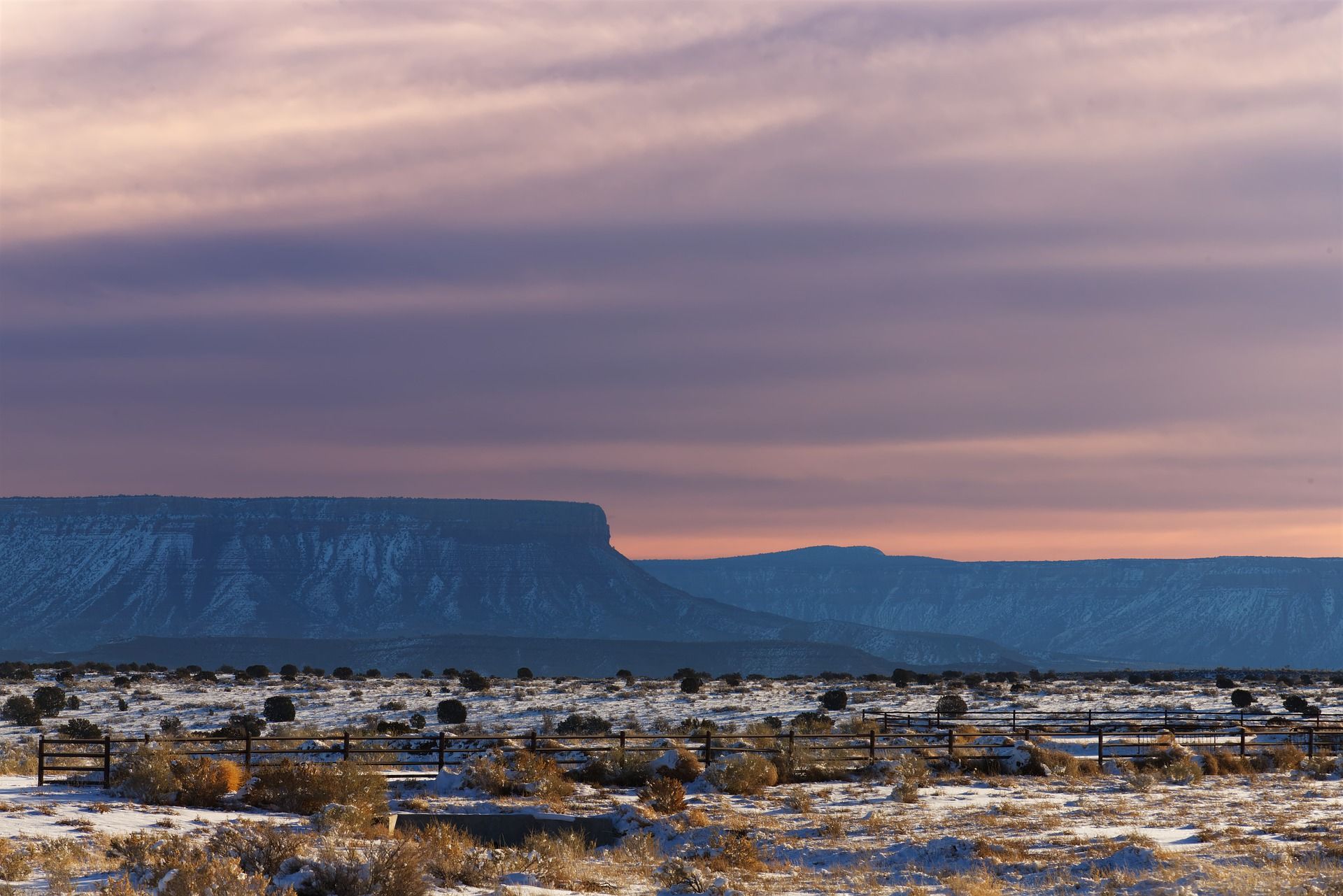
To start out I have figured out the overlap issue with the gecko index by specifying a minimum size of the control. I will have to do this responsively based on viewport size so I should do it in the code for the Gecko Tile.
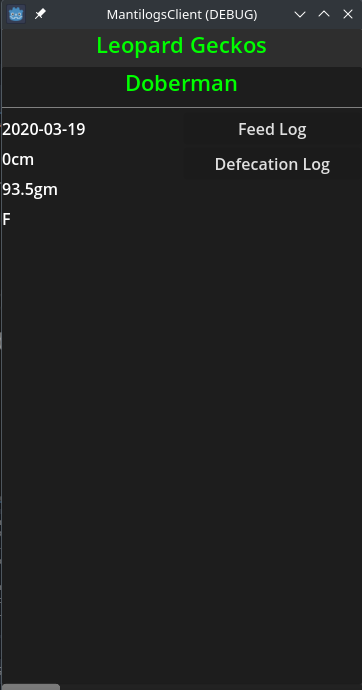
Added some code to the ready function to get the viewport size and scale the tile to it. This will probably look weird on anything but mobile but I will cross that bridge when I come to it. The use-case for this client is mobile for the primary user so that's my focus for now.
Welp.
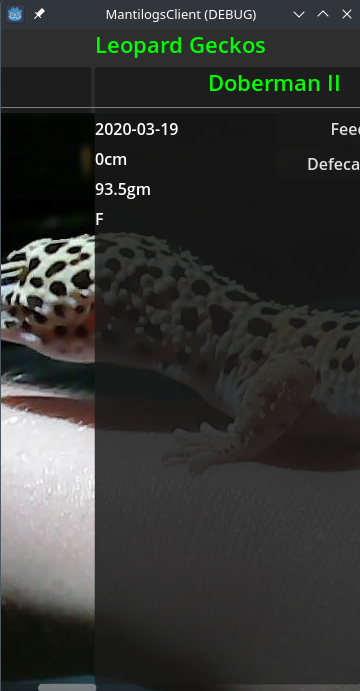
Scaling the images is going to be a thing I have to figure out as texturerect does not obey the rules of size and containers. I will also have to write an adaptive process to handle different formats. Perhaps the data is in the headers and I can use that...

if response_code == 200:
var image = Image.new()
var error
if "Content-Type: image/jpeg" in headers:
error = image.load_jpg_from_buffer(body)
elif "Content-Type: image/png" in headers:
error = image.load_png_from_buffer(body)
elif "Content-Type: image/bmp" in headers:
error = image.load_bmp_from_buffer(body)
elif "Content-Type: image/webp" in headers:
error = image.load_webp_from_buffer(body)
if error != OK:
push_error("Couldn't load the image.")
var texture = ImageTexture.create_from_image(image)
$PanelContainer/VBoxContainer/ProfilePicture.texture = texture
Of course, I probably screwed up the content type headers for a couple, I'll look 'em up... Seems fine from what I see. Alright, now to fix the scaling.
Well, it's all fine and good aside from a couple images leading in upside down for... some... reason.
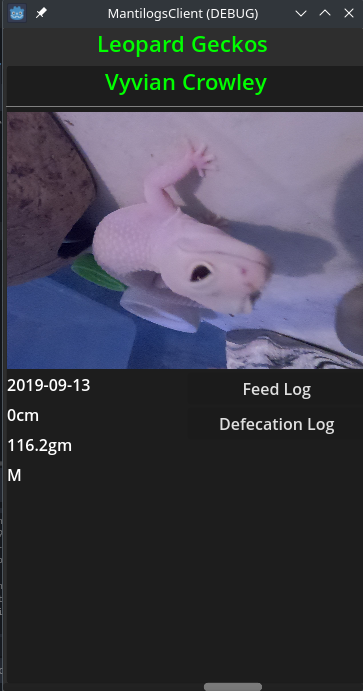
Well, I am sure that the image flippery has to do with the images themselves, I will have to edit them and fix the issues.
I need another end-point, this one for micrologs. I knew I would need more endpoints. I'll leave it alone for now but I have to make an issue so I get to it later.
I also need to add endpoints for cleaning logs. Oh boy. One step at a time, dammit.
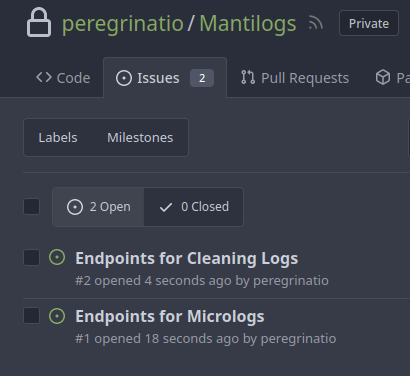
Now back to the task at hand.
I should probably figure out a way to set a maximum height on these texturerects so this doesn't happen;
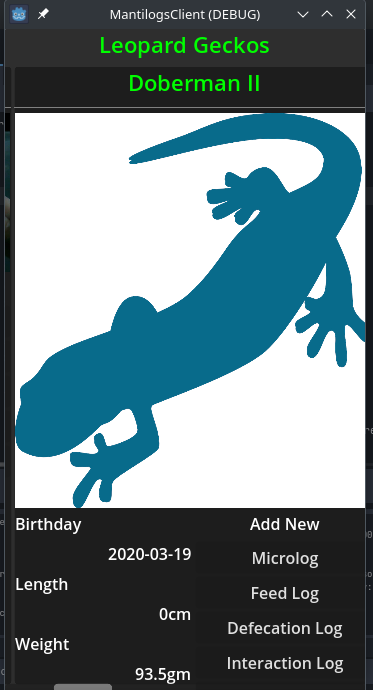
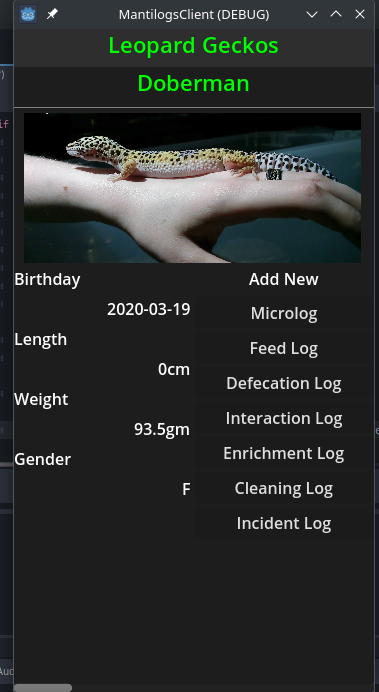
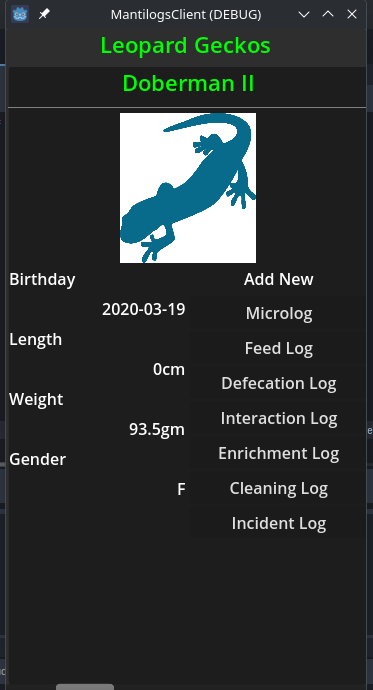
I wish containers had a max size property.
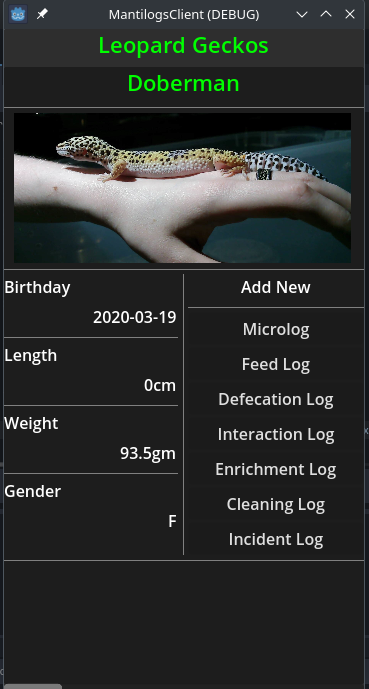
I need yet another endpoint, the needs endpoint. Basically it should look through logs, see when the last time a log was added, compare it to current time and if it is higher than the threshold add it to a list of things needed.
To the issues!
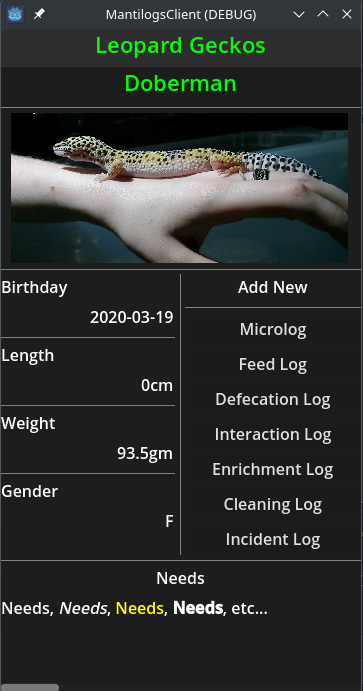
So then, the question I have to ask myself is... do I add the needs endpoint now and finish up this part of the display, then move on to adding the logs and detail view for the geckos or start with adding the logs?
I should probably finish this first before I get on to the next thing. Rather not leave anything half done here.
Added a detail button at the top right, will open a detailed view for the gecko with tabs to check logs and stats.
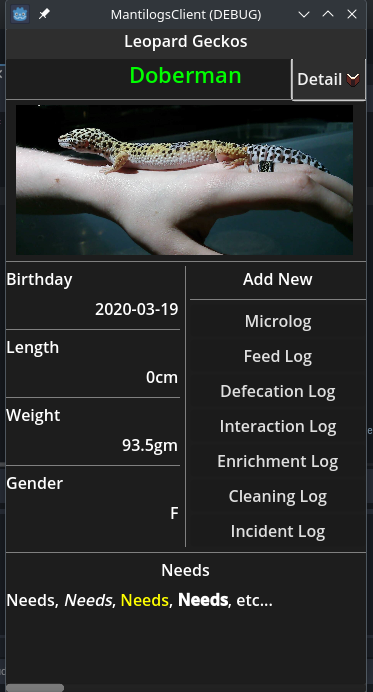
Whoa, 12:15, lunch and shower time.
Much better. Back to work.
def needs_api(request, gecko):
# Get the last log for each category for a particular gecko
# Compare the time on that log against datetime.now
# If the time delta is greater than the threshhold, add it to a dict.
# JSON serialize the dict and return it
needs = {}
#Food
last_feed_logs = Feed_Log.objects.filter(gecko=gecko).order_by('-time')
print((timezone.now() - last_feed_logs.first().time))
print(threshold_feed)
if len(last_feed_logs) > 0:
if (timezone.now() - last_feed_logs.first().time) > threshold_feed:
needs["Feeding"] = timezone.now() - last_feed_logs.first().time
last_calc = last_feed_logs.filter(feed_supplement='CALC')
last_vitd = last_feed_logs.filter(feed_supplement='VITD')
last_mult = last_feed_logs.filter(feed_supplement='MULT')
if len(last_calc) > 0:
if (timezone.now() - last_calc.first().time) > threshold_calc:
needs["Calcium"] = timezone.now() - last_calc.first().time
else:
needs["Calcium"] = "None recorded"
if len(last_vitd) > 0:
if ( timezone.now() - last_vitd.first().time) > threshold_vitd:
needs["VitaminD"] = timezone.now() - last_vitd.first().time
else:
needs["VitaminD"] = "None recorded"
if len(last_mult) > 0:
if (timezone.now() - last_mult.first().time) > threshold_mult:
needs["Multivitamin"] = timezone.now() - last_mult.first().time
else:
needs["Multivitamin"] = "None recorded"
#Water
last_water_log = Water_Log.objects.filter(gecko=gecko).order_by('-time_watered')
if len(last_water_log) > 0:
if (timezone.now() - last_water_log.first().time_watered ) > threshold_water:
needs["Water"] = timezone.now() - last_water_log.first().time_watered
else:
needs["Water"] = "None recorded"
#Moist Hide
last_moist_hide_log = Moist_Hide_Log.objects.filter(gecko=gecko).order_by('-time_moistened')
if len(last_moist_hide_log) > 0:
if (timezone.now() - last_moist_hide_log.first().time_moistened ) > threshold_moist_hide:
needs["Moist Hide Moistening"] = timezone.now() - last_moist_hide_log.first().time_moistened
else:
needs["Moist Hide Moistening"] = "None recorded"
#Tank Clean
tank = Tank.objects.get(gecko=gecko)
last_clean_log = Tank_Cleaning_Log.objects.filter(tank=tank, full_tank_clean=True).order_by('-date')
if len(last_clean_log) > 0:
if (timezone.now() - last_clean_log.first().date ) > threshold_clean:
needs["Tank Clean"] = timezone.now() - last_clean_log.first().date
else:
needs["Tank Clean"] = "None recorded"
return JsonResponse(needs)
There, a needs API. It will return all relevant needs, the thresholds may need tweaking, I will have to ask Moon to give me correct time deltas for each of these.
# Time Delta thresholds for Gecko Needs
threshold_feed = datetime.timedelta(days=7)
threshold_water = datetime.timedelta(days=2)
threshold_moist_hide = datetime.timedelta(days=4)
threshold_vitd = datetime.timedelta(weeks=2)
threshold_calc = datetime.timedelta(weeks=1)
threshold_multivit = datetime.timedelta(weeks=3)
threshold_clean = datetime.timedelta(weeks=4)
I'll likely end up refactoring a lot of things by the end of this month, been working on this thing on and off for 4 years or so, learned a lot in that time that could speed this thing up. But that's for later, for now I need to write the front end portion of the needs API.
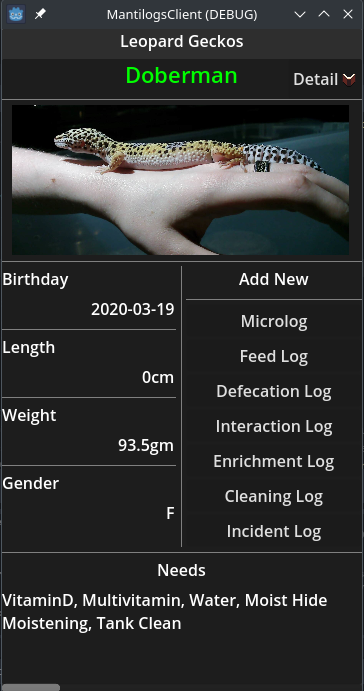
I would like to format them a little better, changing their color depending on how big the delta is but I will do that when I have a clearer idea what the thresholds are.
Now, before I get any further I should probably install android build tools and test this thing on my phone. This will take a minute.
Welp,
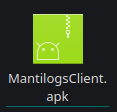
I got a build... now I just have to get it onto my phone. Hmm... web server, perhaps? It's not even configured to look at the right place but I need to see if it even launches to begin with... I guess I can point it at this laptop's IP, should be fine as I bind it to wildcard IP in dev.
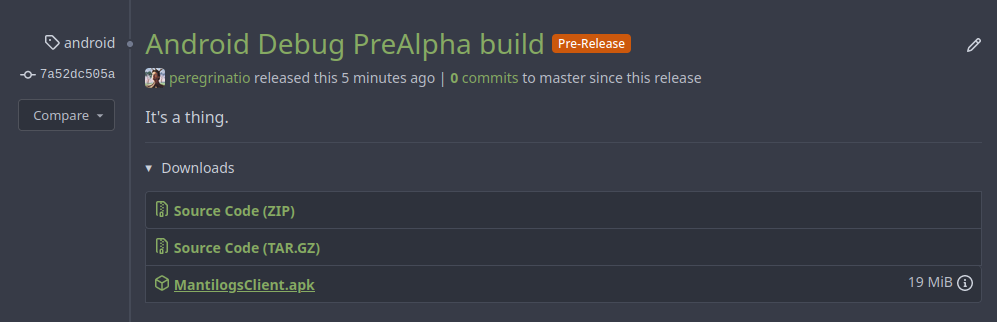
No need for putting it on a local web server after all. Now I will need to login and download it on my phone.
Package appears invalid. Huh, well then.
Manually signed it, maybe that will work?
Yep, can't connect though. Gonna take some work I think... but it's 5pm now so I am clocking out. This is a problem for tomorrow.