Tank work continues
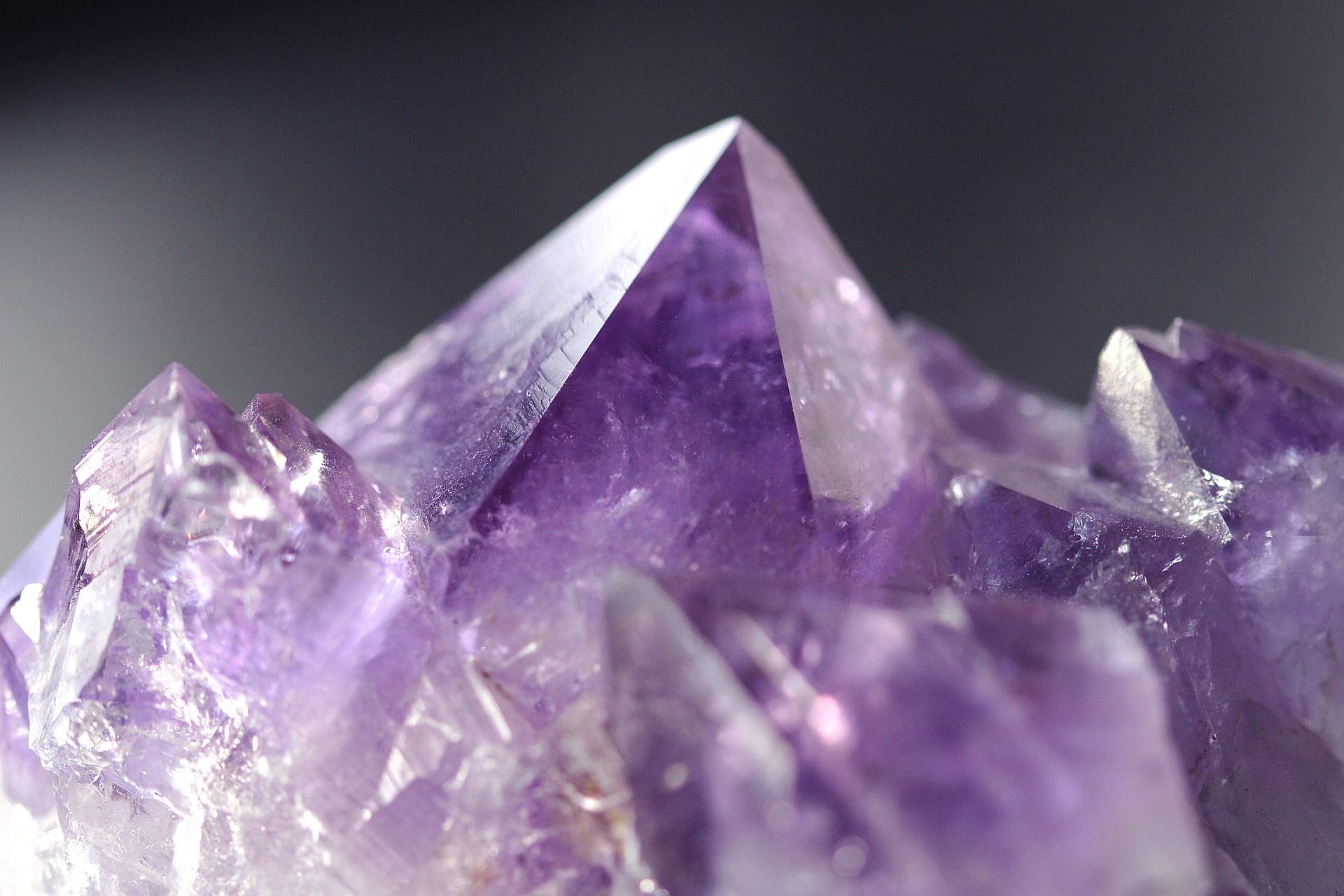
The arm is feeling pretty good today, so that bodes well. We'll see if I am back to a full day or if I need to cut it short again. Gonna play it by ear. It's looking like I just pulled a muscle at this point and that would be nice.
Music for today starts here and continues down the rabbit hole.
Today I will be continuing where I left off yesterday, fixing the bug relating to the tank generation and decor generation not being copacetic. If I write the code in a way that the function waits for a return from the RDBMS before creating the decor item in inventory and placing it I should be alright IF in fact that is the problem. I don't have the same issue with the Apartments which are generated in the same way. Wonder why...
I'll inspect my code for apartment generation in the DBMan and see if there's something I did differently and forgot somehow. I don't think so but it doesn't hurt to check. If that doesn't pan out I will rewrite the generator function to wait for the tank to finish generating and return, then create the object and place it.
It's strange, though. I don't see any async code anywhere in the PostgreSQL client I am using. I may have only looked for some key words that denote an async function or a sub-process or a secondary thread, but I really don't think the code is async so I am not sure why the code creating the decor item would tell me I don't have an object matching the foreign key id in the tank table. This is a strange bug but I will figure it out.
Well, it occurred to me that a refactor may be wise, changing the owner of the tank decor to the apartment rather than the tank itself, this way I don't need to worry about transfer of ownership just to move an object from one tank to another, just what tank something is currently assigned to.
Not sure why I would have wanted to have the tanks have individual inventories really. Probably autopilot mode due to lack of sleep. I really should sort this sleep issue, making lots of damn fool decisions.
I guess I will rewrite the decor_inventory_item to and decor_placement to track what tank something is placed in and set the inventory to be part of the apartment like a less stupid person might have done. Sometimes I just want to take my brain out of my skull and clobber it with a bat.
Looks like I already track the tank, seems like I was going to do it this way at first and probably got distracted. Fixing it now.
Oh, I did do the whole thing like that. What the fuck was I thinking yesterday then? GOD DAMN IT, BRAIN! Get your shit together, man!
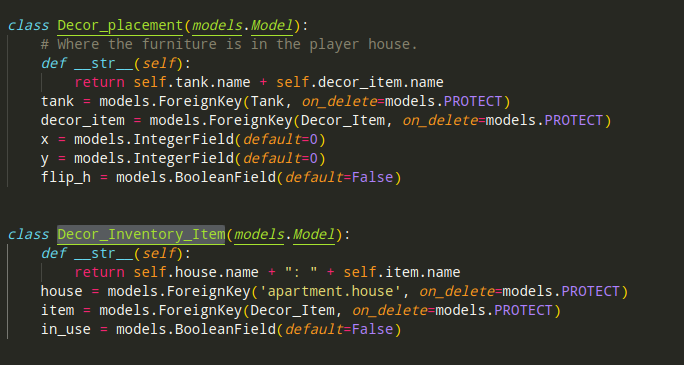
I knew it wasn't async!
OH FOR THE LOVE OF
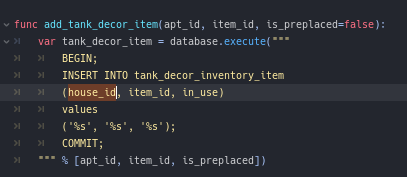
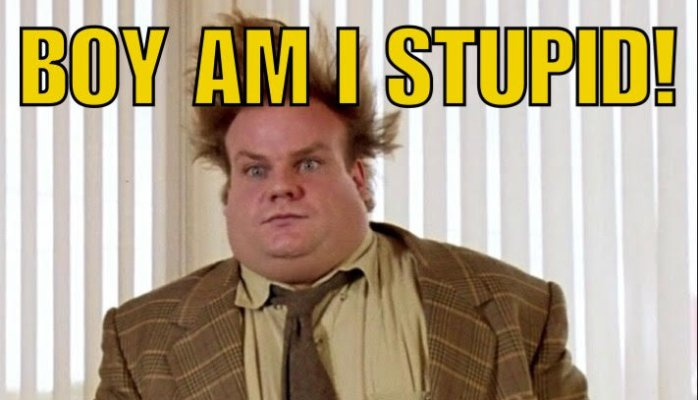
Time to repair stupidity.
func add_tank_decor_item(apt_id, item_id):
var tank_decor_item = database.execute("""
BEGIN;
INSERT INTO tank_decor_inventory_item
(house_id, item_id, in_use)
values
('%s', '%s', '%s');
COMMIT;
""" % [apt_id, item_id, false])
func place_tank_decor_item(apt_id, tank_id, item_id, x, y, flipped):
# Check item is available to be placed, then place it.
if not check_decor_available(apt_id, item_id):
return false
var placed_item = database.execute("""
BEGIN;
INSERT INTO tank_decor_placement
(tank_id, decor_item_id, x, y, flip_h)
values
('%s', '%s', '%s', '%s', '%s');
COMMIT;
""" % [tank_id, item_id, x, y, flipped])
# Set item's in_use bool to true.
var free_item = database.execute("""
BEGIN;
SELECT id FROM tank_decor_inventory_item
WHERE in_use = '%s' AND house_id = '%s';
COMMIT;
""" % [false, apt_id])
var free_item_id = free_item[1].data_row[0][0]
var placed_item_inv = database.execute("""
BEGIN;
UPDATE tank_decor_inventory_item
SET in_use = '%s'
WHERE id = '%s';
COMMIT;
""" % [true, free_item_id])
return true
Now lets see how this breaks.
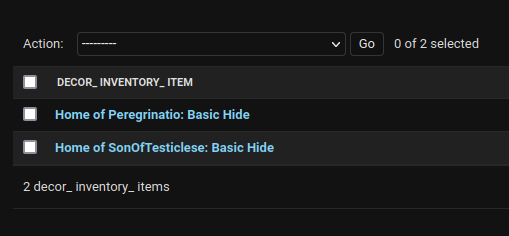
Now I need to figure out why I am having a problem with placement.
First thought is the inventory ID is returning wrong.

So the stupid of yesterday has continued into today.


Now let's make sure everything is where it should be.
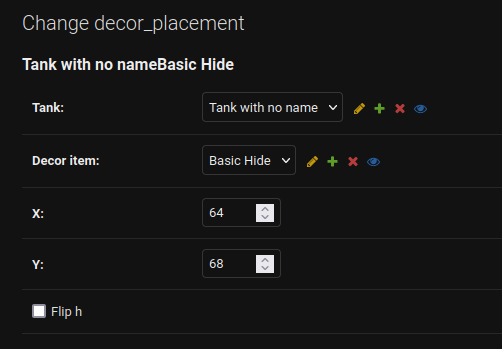
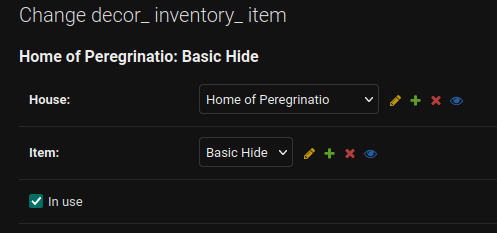
Looks like I got it working now.
Now on to the client side and sending the data over, displaying it... all that. A bit overwhelming really. One step at a time.
- Create a Tank Scene
- Create a UI element to display the Tank Scene in (Using Viewport?)
- Make Tanks placeable in Apartment, make sure they keep track of their own ID. Have their interact() open the UI element above.
- Create a Tank Decor Inventory inside the Tank Scene.
- Send Tank Data and Decor Inventory Data to Client.
- Place Tank Decor Items in Tank Scene.
- Create Tank Inventory UI in Tank Scene.
- Populate Tank Inventory with relevant data (Things not in_use).
- Create Placement and Removal functions for Decor.
- Make sure inventory updates accordingly with placement and removal of Decor.
- Make absolute certain that there is no way the server can tell the DB to place an item that doesn't exist in the player's apartment inventory and there is no way to place an item from one apartment's inventory in another apartment's tanks.
- Create a Tank decor item with a server side script and make sure it loads and runs correctly (Really just needs to be a print statement for now).
There's probably going to be a bit more to it than that but that should be a good overview to get me going.
I already made a basic tank from the prototyping phase before I started all this network code. I'll dupe it and start there.
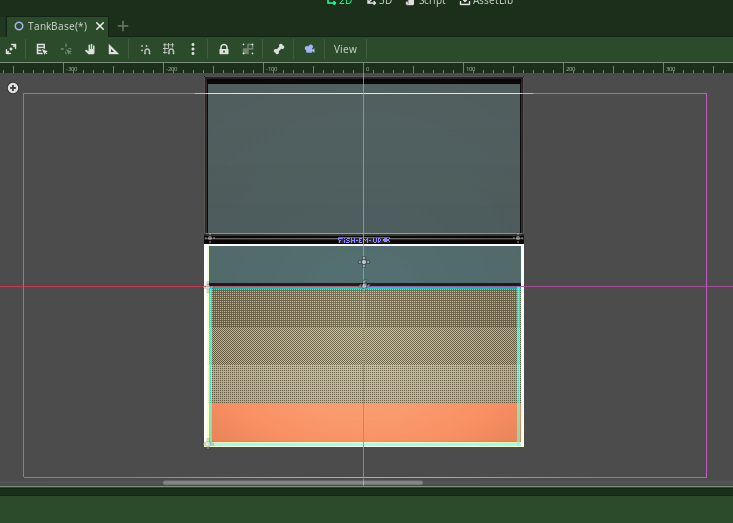
Next up... cramming it into a UI element.
Oh, thankfully the great people developing Godot have already considered this.

Now to actually center the tank.
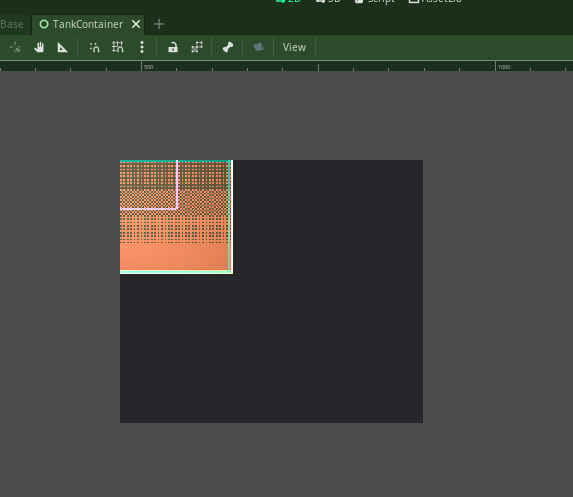
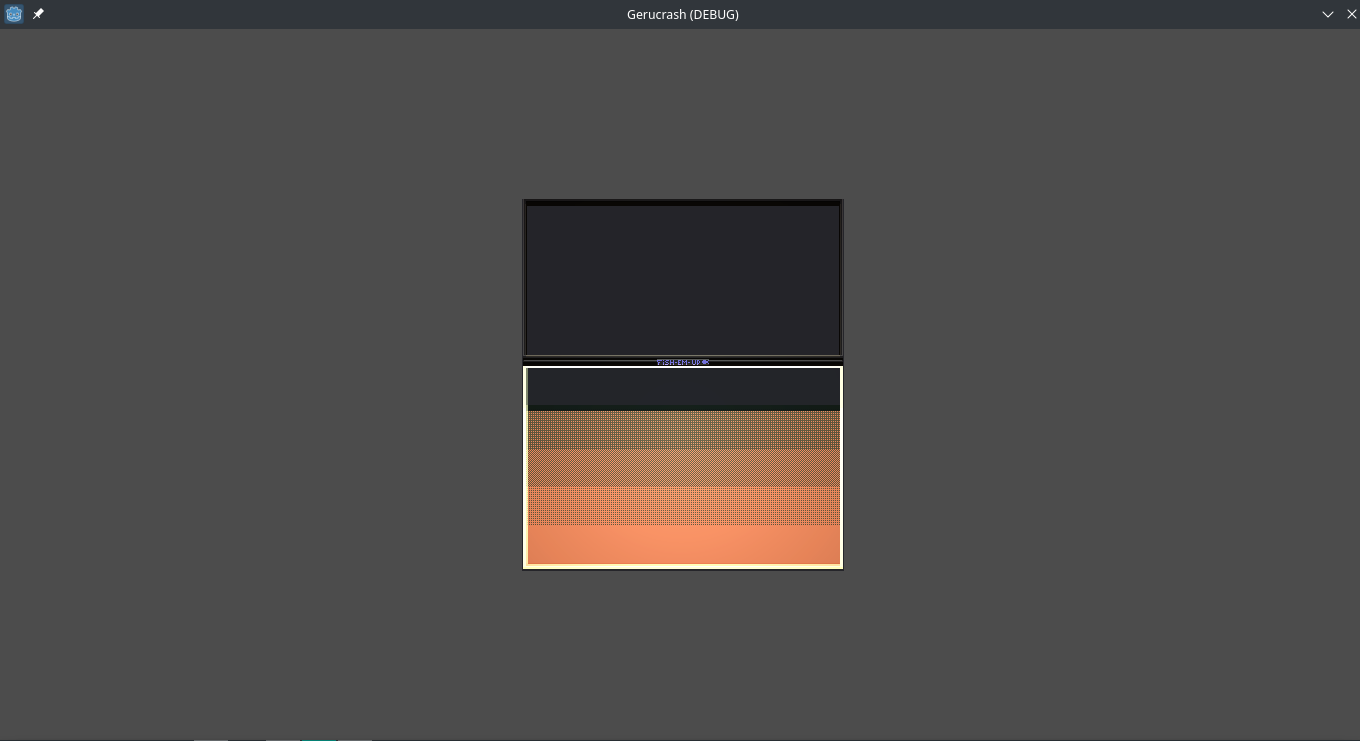
Next I will need to add some kind of indication that this thing is a window.
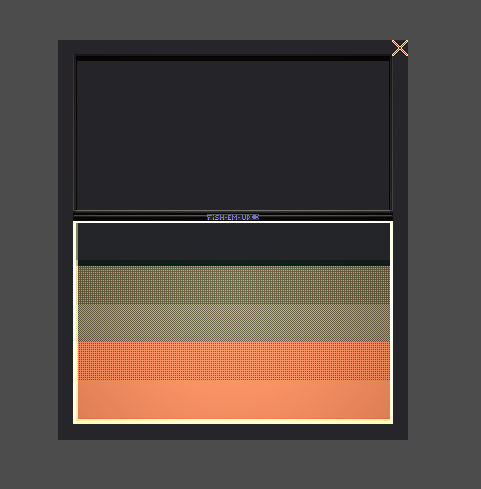
Alright, the background of the panel can be a customizable thing later, for now it'll be basic, standard, dark grey. Fine for now, now is not the time to make things pretty, now is the time to make things work.
Well, that's #2. 3 will take a little more work.
I'll need a sprite for the tank, I have one somewhere here that I made in the early prototyping phase, I should be okay to use that. The furniture item when clicked will fire the interact() function, if there is one and in there we will populate and display this little panel.
So I should create a furniture item for the BasicTank and then there's the whole populating it as part of the inventory bit, then we'll pick up from there.
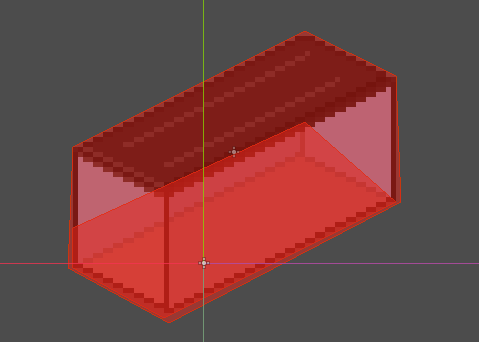
Lunch must be made. Then the placement of the tank will begin.
To place a tank I think I will create a secondary inventory in the apartment on the server because I will be using a slightly different data structure and it would be cheaper on the hardware to not have to test every single thing in an array to see if it is a tank. Also going to have a second furniture placement array for tanks, same reason. They need to have an ID generated on load to make them identifiable.
I don't want to send the actual ID to the client, I don't trust the client. I will use a new UUIDv4 that will resolve to the real ID server-side. Or I could use an Incremental ID, which would be faster, probably. UUID generation is a bit slower than figuring out how many tanks there are in the apartment and adding one.
I would have to set their names based on the new ID...

This will allow me to hide the tank ids from the client and not have to deal with any extra overhead.
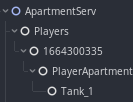
Now I need to get the tank items from the DBMan into the new Tank inventory.
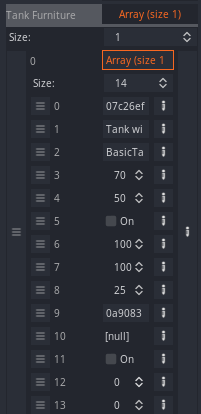
I'll replace that first value(id) with the new ID (Tank_#) for each tank as I process them so when I send them over to the client there's no ID available. It will still have the Geru ID attached for now but there's no Geru yet so... meh? I will make an issue for that though, so I don't forget.
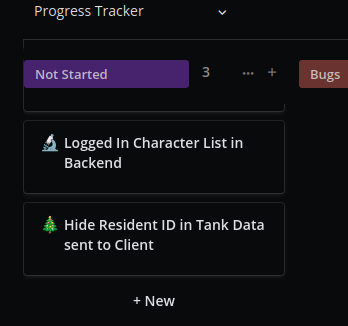
Now I have the data and am ready to send it over to the client... I created another tab in the inventory for tanks so once I have the data I can populate it with their data.
I keep feeling overwhelmed today, There's a lot going on with this project and a lot to keep track of. That's kinda what this whole log is about, helping me sort it all out and keep track of things.
So first I need to make sure I am receiving the data correctly and then I can populate my inventory tab with it.
Now I need to remember where in the client I call the code to populate the inventory UI. Hmm...
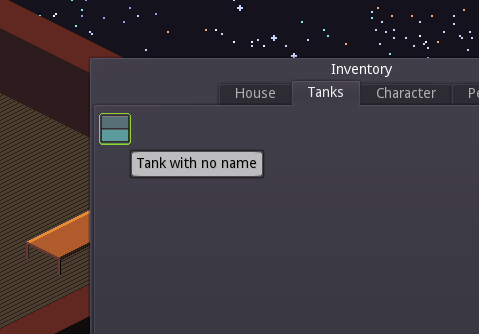
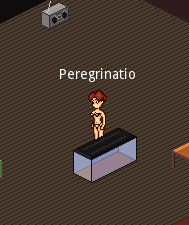
Now I just need to make a placement scene for it, since the furniture one isn't set up to handle tank data. Though I can just copy the furniture one and modify it to save myself a lot of time.
So now the button needs to pass the tank_id to the placement scene, which then has to pass it to the furniture_item.
Whoa, really cloudy mind suddenly. Maybe lunch? Maybe need to take a short break? I'll take a short break I guess, might clear my head and allow me to focus on this a little better.
I wonder why I didn't just set the placement data on the furniture inventory? I probably had a reason but I don't know what it was. Anyway, I may refactor that later if I can't remember why. So with tanks I will do it that way, I could use furniture placement but I feel like separating them and trying a new way will teach me more and help me decide if I want to refactor furniture placement later.
I need to add a flipped field to the tanks though, already have the X and Y but for some reason didn't bother with the flipped bool. This will take a little refactoring in the server and model. Should be fairly straightforward and quick, though. (Should.)
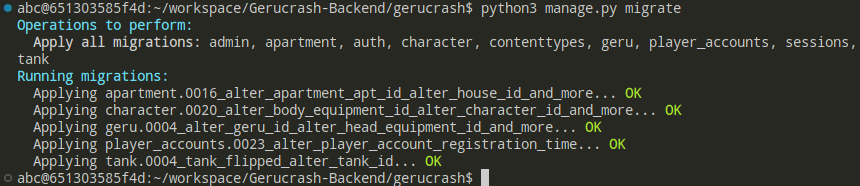
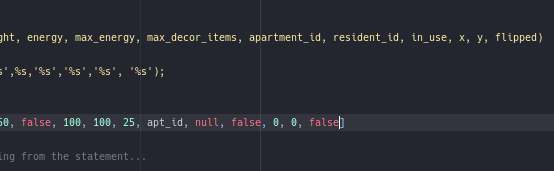
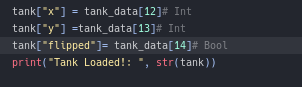
Should be all I need to do...
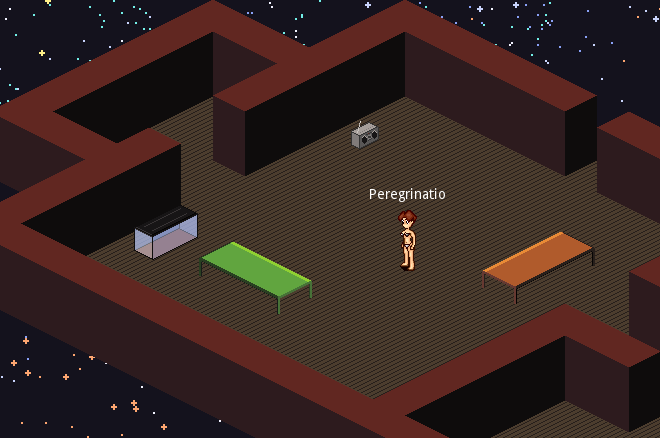
It looks like it worked but... hmm... let's find out.
Of course not, had a field wrong in the query there, but hey, better than usual.
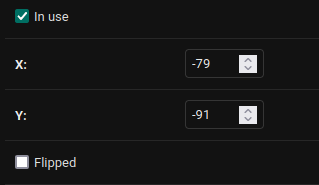
So we're able to place it. I still have to write the code to load it in when a player logs in, but that was way quicker than furniture placement. Standing on the shoulders of... me... last week.
Alright, guess I better write that code in that loads the tanks when the player logs in, eh?
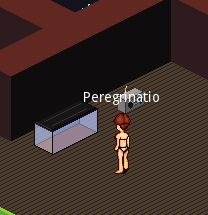
Will it be there?
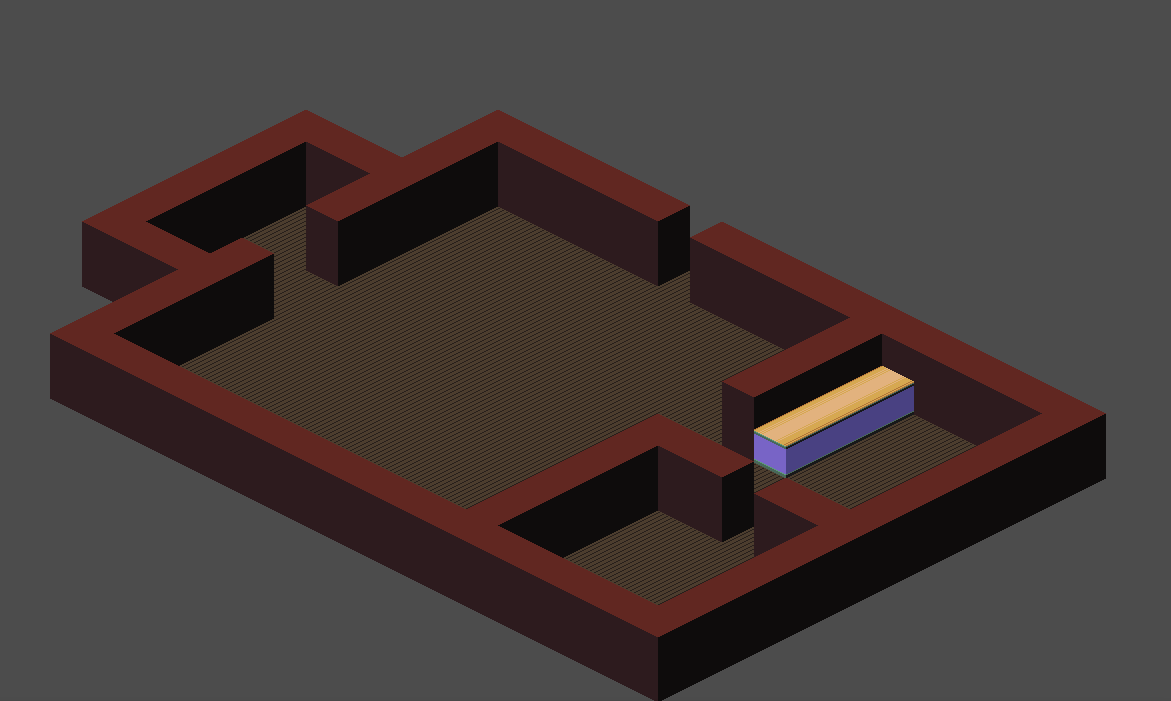
I put a dot where I wanted a slash, what a fool.


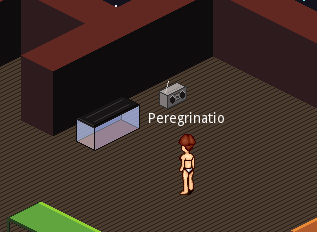
Alright, now to make sure the tank gets it's data correct on loading, because right now we got a blank ID.

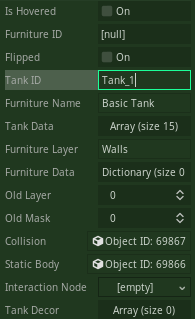
Next I need to retrieve tank decor placement, build a UI for decor inventory that will exist inside the tank scene and pop the viewport UI open whenever the interact is fired.
I've got it scaffolded for tomorrow because my arm is starting to get a bit of an ache now, made it to 4pm today so it is definately improving. Maybe tomorrow I will actually get a full day in.
I did manage to accomplish quite a bit today, it's only Tuesday and I expected it to take me until the end of tomorrow or halfway through Thursday to be where I am so I don't feel so bad about knocking off early.
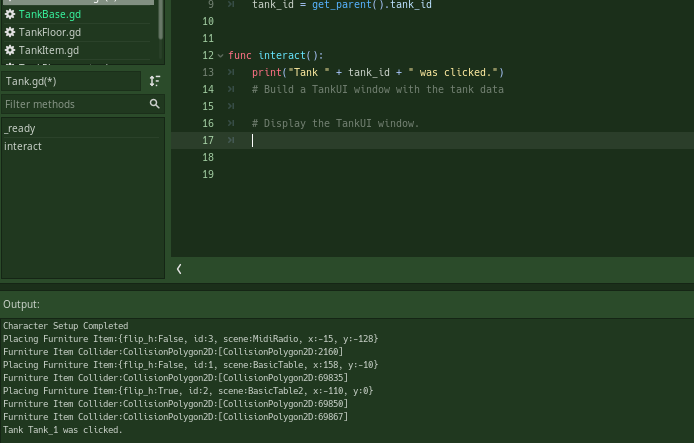
I also added a call to the load_decor() func in the tank from the apartment when it's loading the tank initially. It will request the server send it's decor data.
Anyway I am out of here, gotta rest my arm and make some dinner in a bit. Tomorrow I will pick up here and maybe by the end of the day have decor placement handled client-side and the ability to pick up and put down decor. Maybe. I still need to make the decor inventory and get the decor loading from the server and all that.